Update: There seems to be a bug in ExpandAssetCategoryId
as I can retrieve all the assets (even those in custom DBs) if I start from a manually generated list of category Ids, but I only geta couple of the categories using this method.
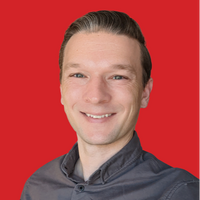
Posts made by d_keith
-
RE: Traversing Asset Categories
-
Traversing Asset Categories
Hi @ferdinand - Thank you so much for this!
This works great for Maxon's built-in assets databases. How would I retrieve all assets that belong to a category, even if they live in custom user-mounted databases?
GetUserPrefsRepository()
seems like the best bet, but it doesn't seem to include the many assets I have in custom DBs, and I don't see any other promising methods exposed in the SDK.edit: @ferdinand - Forked from Get All Assets in Category, although the other thread was named appropriately, it was more about general asset traversal and not the specific makeup of asset category trees.
-
RE: Get All Assets in Category
Prints a list of Media Assets in the Cinema 4D Assets Browser to the Console [GitHub Gist]
"""Name-en-US: Print Media in Category Description-en-US: Prints a list of all media assets belonging to a category ID to the console. References: https://developers.maxon.net/forum/topic/14214/get-asset-from-asset-browser-python/4 ## License MIT No Attribution Copyright 2022 Donovan Keith Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. """ # Imports from importlib.metadata import metadata from multiprocessing.sharedctypes import Value import c4d import maxon from typing import Optional # Helper Functions def FindAssetsByType(type) -> Optional[list[maxon.Asset]]: repository = maxon.AssetInterface.GetUserPrefsRepository() if not repository: raise RuntimeError("Unable to get User Repository.") # Find Assets: # https://developers.maxon.net/docs/py/2023_2/modules/maxon_generated/frameworks/asset/interface/maxon.AssetRepositoryInterface.html?highlight=findassets#maxon.AssetRepositoryInterface.FindAssets assets = repository.FindAssets( assetType=maxon.AssetTypes.File(), aid=maxon.Id(), version=maxon.Id(), findMode=maxon.ASSET_FIND_MODE.LATEST, receiver=None ) return assets def FindFileAssets(): return FindAssetsByType(type=maxon.AssetTypes.File()) def GetAssetCategory(asset: maxon.AssetInterface): if not asset: raise ValueError("No asset provided.") meta_data = asset.GetMetaData() if not meta_data: raise ValueError("Unable to get asset meta data.") return meta_data.Get(maxon.ASSETMETADATA.Category) def GetAssetName(asset: maxon.AssetDescription) -> Optional[str]: if not asset: return metadata: maxon.AssetMetaData = asset.GetMetaData() if metadata is None: return name: str = asset.GetMetaString( maxon.OBJECT.BASE.NAME, maxon.Resource.GetCurrentLanguage(), "") return name def IsAssetAnImage(asset: maxon.AssetDescription) -> bool: if not asset: return metadata: maxon.AssetMetaData = asset.GetMetaData() if metadata is None: return sub_type: maxon.Id = metadata.Get(maxon.ASSETMETADATA.SubType, None) if (sub_type is None or maxon.InternedId(sub_type) != maxon.ASSETMETADATA.SubType_ENUM_MediaImage): return False return True def GetCategoryIdFromUser() -> str: imperfections_id_string = "category@e780d216ed404547942dcbfcbbe009e5" category_id_string = c4d.gui.InputDialog( "Input Category ID", preset=imperfections_id_string) if not category_id_string: raise ValueError("Invalid ID String") return maxon.Id(category_id_string) def main(): category_id = GetCategoryIdFromUser() file_assets = FindFileAssets() imperfections_assets = [asset for asset in file_assets if ( IsAssetAnImage(asset) and GetAssetCategory(asset) == category_id)] for asset in imperfections_assets: print(GetAssetName(asset)) if __name__ == "__main__": main()
-
Get All Assets in Category
Question
How can I retrieve all image assets that live in a given category (and sub-categories) in the assets browser?
Ideally, I could use something like Python glob syntax:
glog.glob("./Textures/Imperfections/**/*.png")
And get back a list of assets
[ "si-v1_deposits_01_15cm.png", "si-v1_desposits_02_15cm.png", ... ]
Related
- Asset Metadata - Search for Asset Categories by Path : Cinema 4D C++ SDK
- Can I download a Tex in Asset Browser and add into select RS node mat? | PluginCafé
- Get asset from asset browser python | PluginCafé
- Get All Assets in Category | PluginCafé
- Asset API : Cinema 4D C++ SDK
- cinema4d_py_sdk_extended/scripts/05_modules/assets at master · PluginCafe/cinema4d_py_sdk_extended · GitHub
-
RE: Looking for Scene Nodes Capsules Creators
Hi All,
Thank you so much for your interest and taking the time to apply. We ended up with more applicants than could fit in one workshop. If you received an email from me (Maybe in your spam folder? look for
d_keith
) you've likely been accepted. If not, I do intend to host similar workshops in the future. Candidates were given priority based on:- Prior programming expertise in C/C++/Python or similar languages.
- Interest and ability to actively participate in the lectures/homework projects.
- Availability to potentially create capsules for the Maxon Capsules Library in the future on a freelance basis.
- And for auditors, special preference was given to MAXON employees who will be documenting/developing/training Scene Nodes workflows.
I hope to host similar workshops in the future and will prioritize previous applicants at that time.
Sincerely,Donovan Keith
-
RE: Looking for Scene Nodes Capsules Creators
Thanks all for your replies! I'll be reviewing this week and will target a July 1 start.
-
Looking for Scene Nodes Capsules Creators
Hi!
I'm a Product Manager and Assets Producer at Maxon. We recently collaborated with the team at Rocket Lasso to produce some great new FUI Spline Capsules (
, & ) and we're hoping to replicate that success with additional third party partners.Are you a plugin developer or technical director? Have you been interested in Scene Nodes, but not sure where or why to start?
We're looking to grow the number of qualified C4D Scene Nodes capsule creators, and improve scene nodes workflows in Cinema 4D. To that end, we're training/supporting a small group of interested developers/technical directors over a ~4 week period. If you're interested in participating, please fill out this form before 2022-06-20:
https://forms.office.com/r/Mqyq37fXST
Specifics will largely depend on the group we select, but we're looking to provide:
- Live weekly video training/Q&A sessions (Time TBD, but probably Friday nights in Europe / Friday mornings in the US), with possible supplemental pre-recorded videos.
- Best practices documentation.
- Direct access to a Maxon product manager.
- Suggestions for how to improve your node setups and UX to match C4D performance/UX standards.
- A private forum for discussing ideas/issues with other Scene Nodes Assets creators.
- A private repo of utility node groups for solving common issues.
You'll be asked to:
- Complete 1+ Spline/Geo Primitive, Geo Modifier, Selection Modifier, or Node Group that feels and functions like a native Cinema 4D primitive/deformer/node.
- Document your development process.
- Provide honest feedback about Scene Nodes workflows and documentation.
- Log any bugs/UX issues you encounter as you work.