Hi all, my first post here. Thank you for the opportunity.
I'm developing a C++ ObjectData plugin. During testing it seems that straight from the start multiple instances of the plugin are created. Why is that and can it be avoided?
I've cobbled together a bare bones plugin (called Minst, plugin ID=1111111) which displays the same behaviour as my actual plugin.
Here's the output to the Console:
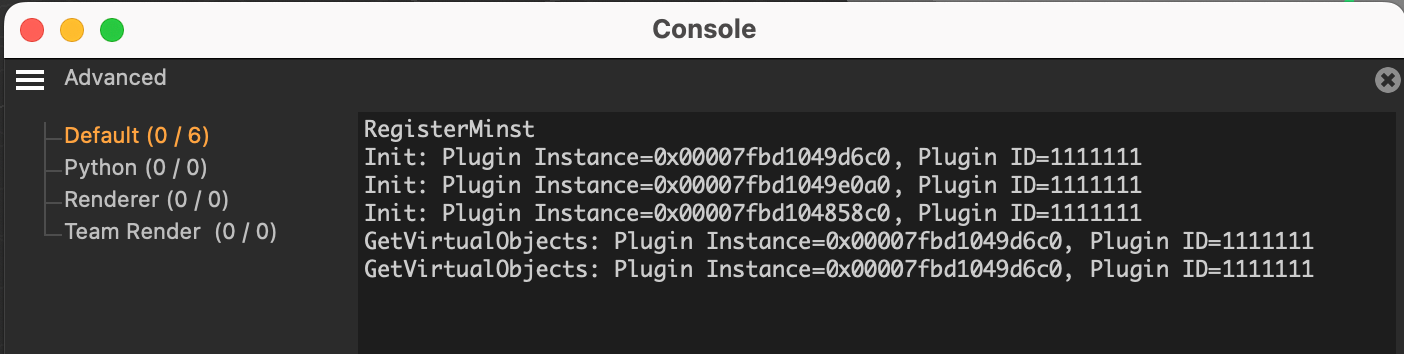
I believe the Console shows that multiple instances of Minst are created by calling Init() more than once. (My more complex plugin also calls GetDDescription() more than once, so this multiple calling of the same functions can become quite involved very quickly. That's why I would very much like to know the reasoning behind it.)
The Console also shows that GetVirtualObjects() is only called for the first instance of the plugin, not the other two, which seems a good thing. (However, it also shows that GetVirtualObjects() is called twice in a row, one right after the other, which seems a bit superfluous.)
Here 's the code:
main.h
#ifndef MAIN_H
#define MAIN_H
Bool RegisterMinst();
#endif
main.cpp
#include "c4d.h"
#include "main.h"
Bool PluginStart()
{
return RegisterMinst() != 0;
}
void PluginEnd()
{
}
Bool PluginMessage(Int32 id, void* data)
{
switch (id)
{
case C4DPL_INIT_SYS:
{
return g_resource.Init() != 0;
}
case C4DMSG_PRIORITY:
{
return true;
}
default:
return false;
}
}
minst.cpp
#include "c4d.h"
#include "main.h"
#define ID_MINST 1111111 // Temporary plugin id. Replace this with a proper ID.
class Minst : public ObjectData
{
INSTANCEOF(Minst, ObjectData)
public:
Bool Init(GeListNode* node) final;
BaseObject* GetVirtualObjects(BaseObject* op, HierarchyHelp* hh) final;
Bool Message(GeListNode* node, Int32 type, void* t_data) final;
static NodeData* Alloc() { return NewObjClear(Minst); }
};
Bool Minst::Init(GeListNode* node)
{
maxon::String message = FormatString("Init: Plugin Instance=0x@, Plugin ID=@", (void*)this, node->GetType());
ApplicationOutput(message);
return true;
}
Bool Minst::Message(GeListNode* node, Int32 type, void* t_data)
{
return true;
}
BaseObject* Minst::GetVirtualObjects(BaseObject* op, HierarchyHelp* hh)
{
maxon::String message = FormatString("GetVirtualObjects: Plugin Instance=0x@, Plugin ID=@", (void*)this, op->GetType());
ApplicationOutput(message);
return nullptr;
}
Bool RegisterMinst()
{
ApplicationOutput("RegisterMinst");
return RegisterObjectPlugin(ID_MINST, "Minst"_s, OBJECT_GENERATOR, Minst::Alloc, ""_s, nullptr, 0);
}
Can someone please shed some light on this?
Thank you.