@ferdinand
Thank you Ferdinand for explaining everything!
Currently, I decided to temper with the normals of my polygon object to make smoothing by hand.
However, what I noticed, is the following:
Suppose I don't create normal tag and simply create a phong tag for my polyobject.
Unfortunately, despite appearing in the menu of the object, somehow it doesn't work (despite my changing the phong angle in the menu).
At the moment I created a function which simulates the extrude functionality (for the reasons of plugin, I refrain from using the built in c4d extrude function )
I feed it with initial closed spline, the extrude height and precision (The way I interpolate curved elements of the spline with line elements)
void wall(BaseDocument* document, SplineObject* spline, double height, double precision) {
LineObject* spline_linear = spline->GetLineObject(document, precision);
int n1 = spline_linear->GetPointCount();
Vector* gp = spline_linear->GetPointW();
// define point and polygon count
const Int32 polyCnt = n1;
const Int32 pointCnt = 4 * n1;
// create polygon object
PolygonObject* const polygonObject = PolygonObject::Alloc(pointCnt, polyCnt);
// insert object into the document
document->InsertObject(polygonObject, nullptr, nullptr);
// access point and polygon data
Vector* const points3 = polygonObject->GetPointW();
CPolygon* const polygons = polygonObject->GetPolygonW();
for (int i = 0; i < n1 - 1; i++) {
// set points
points3[4 * i] = gp[i];
points3[4 * i + 1] = gp[i + 1];
points3[4 * i + 2] = Vector(gp[i + 1].x, 100, gp[i + 1].z);
points3[4 * i + 3] = Vector(gp[i].x, 100, gp[i].z);
// set polygon
polygons[i] = CPolygon(4 * i, 4 * i + 1, 4 * i + 2, 4 * i + 3);
}
points3[4 * (n1 - 1)] = gp[n1 - 1];
points3[4 * (n1 - 1) + 1] = gp[0];
points3[4 * (n1 - 1) + 2] = Vector(gp[0].x, 100, gp[0].z);
points3[4 * (n1 - 1) + 3] = Vector(gp[n1 - 1].x, 100, gp[n1 - 1].z);
// set polygon
polygons[n1 - 1] = CPolygon(4 * (n1 - 1), 4 * (n1 - 1) + 1, 4 * (n1 - 1) + 2, 4 * (n1 - 1) + 3);
BaseTag* phongTag = polygonObject->MakeTag(Tphong);
polygonObject->Message(MSG_UPDATE);
}
I wonder What has gone wrong?
The thing builds nice polygonal object, but it is not smooth! ![alt text]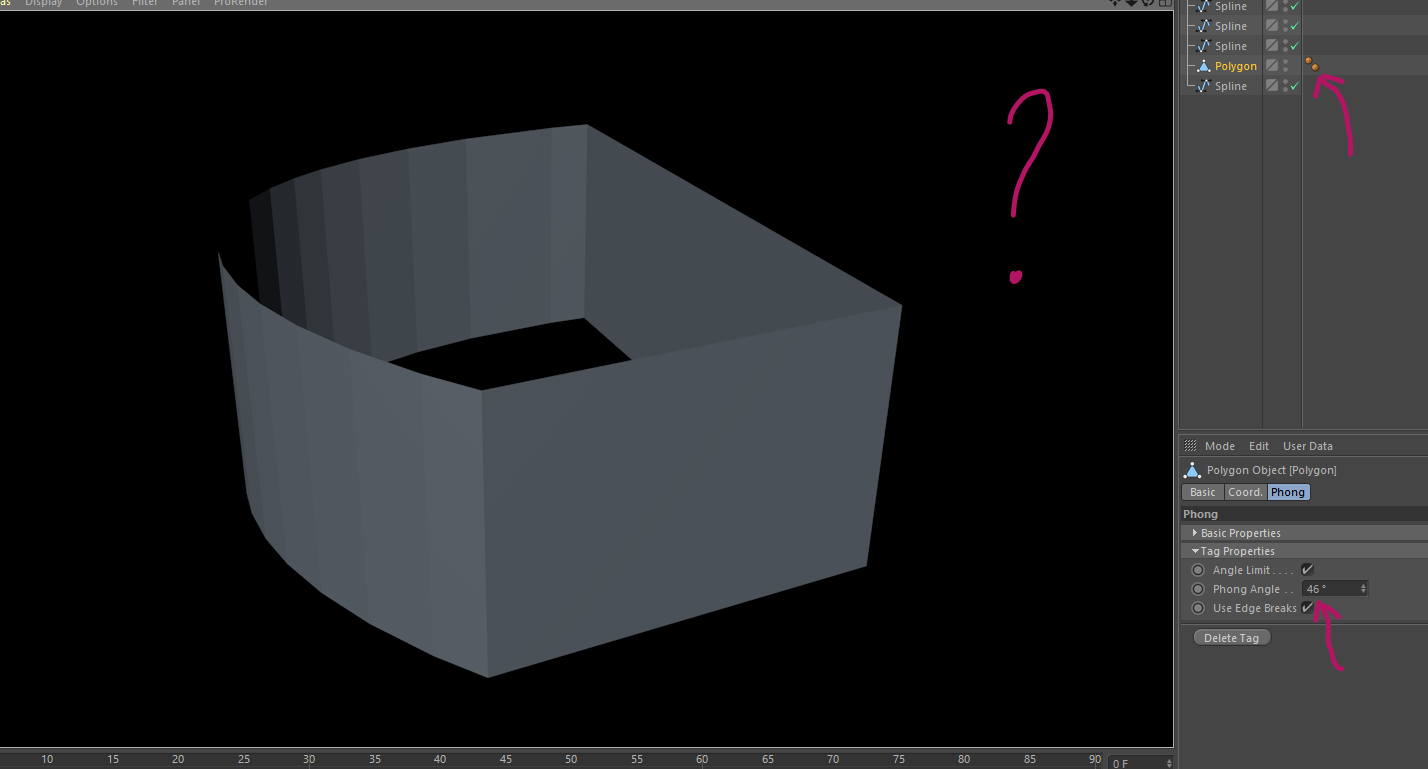