Python Script Manager¶
Introduction¶
The Script Manager can be found in the Script Menu -> Script Manager.
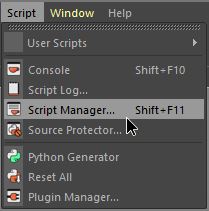
In the Script Manager, Python code can be written, executed, saved, imported, exported.
By default, there is no script loaded in the Script manager. To create a new script go to File Menu -> New. This will create a new script with a default code.
Since scripts are not automatically saved, it’s mandatory to either press CTRL+S (Windows) or CMD+S (Mac Os) while the Script Manager Dialog is the active one. It’s also possible to save through the File Menu -> Save of the Script Manager.
All saved or imported script will be located in {C4D_PREF_FOLDER}\library\scripts\ folder. If a file is not saved a * will be present at the end of the filename within the drop-down list.
Note
{C4D_PREF_FOLDER} can be found by opening Cinema 4D Preference (CTRL+E) and by clicking into the button “Open Preference Folder…” in the bottom of the dialog.
On Windows, a local or a network path containing other scripts that will be load on startup can be defined. Sets an Environment Variable named {C4D_SCRIPTS_DIR} and defines the path like c:\my_c4d_scripts
The Script Manager can also be used to retrieve Symbol ID for Parameters. See Drag And Drop.
Features¶
Code Execution¶
The code run from the Script Manager is executed in Cinema 4D main thread. This means that if an infinite loop takes place, Cinema 4D will hang as well. See Threading Information.
It’s possible to Execute code by pressing the Execute button in the bottom of the Script Manager or by transforming the Script into a Button. See Button.
By click on the Shortcut… button it’s possible to assign a shortcut to the script. Each time the shortcut is pressed the script will be executed.
Variables¶
- Next variables are pre-defined in the Script Manager:
doc:
BaseDocument
: The current active document. Similar toc4d.documents.GetActiveDocument()
.op:
BaseObject
: The current active object. Can be None if no object is selected. Similar toBaseDocument.GetActiveObject()
Button¶
It’s possible to transform a script into a button by drag and dropping the script icon to somewhere in the UI.
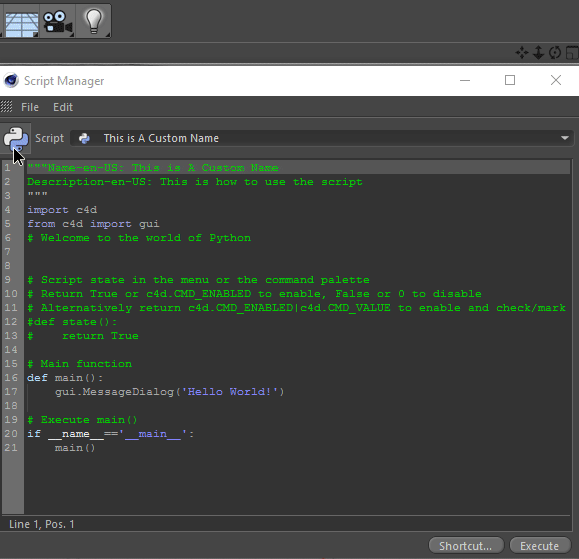
All python scripts added to the UI (a button or a menu entry) give the chance to be edited by left-clicking on it and selecting the Edit Script command.
If your script is mainly used as a button, since R20 a function called state can be defined to retrieve if the button should be enabled, disabled or checked.
- The state function can be something like that and expect to return a boolean value or these constants:
True: Enable the button, similar to CMD_ENABLED.
False: Disable the button.
CMD_ENABLED: Enable the button, similar to True.
CMD_VALUE: Check the button.
def state():
# Check if an object is selected.
if op:
return c4d.CMD_ENABLED | c4d.CMD_VALUE
return False
Warning
The state function is called in each UI redraws (multiple time per second), which is executed in the c4d main thread. So try to avoid as much as possible any time-consuming operation to not freeze Cinema 4D.
Customization¶
All scripts loaded in the Script Manager get a default python icon, it’s possible to change this icon with a custom picture from the menu File -> Load Icon….
The name and the description of a script can be changed with the following header comments (FileName will not be changed only display name).
"""Name-en-US: This is A Custom Name
Description-en-US: This is how to use the script
"""
Drag And Drop¶
Parameter¶
All parameters can be dragged to the Script Manager. It allows to set/get parameter. It can also be used to know the symbol of a parameter.
Note
If the Size.X parameter of a cube is dragged into the console, the following text will be printed:
« Cube[c4d.PRIM_CUBE_LEN,c4d.VECTOR_X] »
‘Cube’ is a variable, named as the object it’s refer to. The object is the host of the parameter. This means that if [c4d.PRIM_CUBE_LEN,c4d.VECTOR_X] is removed, writing Cube.GetName() it will returns the name of the object.
- ‘[c4d.PRIM_CUBE_LEN,c4d.VECTOR_X]’ can be splitted in multiple parts:
[] redirect to
GetParameter
orSetParameter
of an object according to the context.c4d.PRIM_CUBE_LEN, is the symbol corresponding to the Size parameter.
c4d.VECTOR_X, is the symbols corresponding to the X parameter of the Size Parameter.
It’s possible to edit the parameter value with this code:
Cube[c4d.PRIM_CUBE_LEN,c4d.VECTOR_X] = 50.0